
Click to see it work
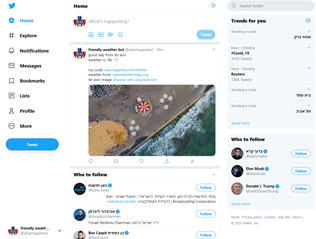
1. gets tel aviv weather from openweathermap
2. gets tel aviv image from unsplash.com
1. gets tel aviv weather from openweathermap
2. gets tel aviv image from unsplash.com
#friendly weather twitter bot
#gets region weather and image, then tweets it
import tweepy, requests, json, time
import keys #file with twitter (an other apps) login keys
place = "tel aviv"
while True:
#get weather from openweathermap
r = requests.get(url="http://api.openweathermap.org/data/2.5/weather?units=metric&q=" +
place + "&units=metric&appid="+keys.openweathermap_app_id)
weather = round(r.json()['main']['temp'])
#convert weather to icon
icon = ''
if (weather <= 14):
icon = '❄'
elif (weather <= 21):
icon = '☁'
elif (weather <= 28):
icon = '🏕'
else:
icon = '🏖'
#get image according to place
r = requests.get(url="https://api.unsplash.com/photos/random?query=" +
place + "&client_id="+keys.unsplash_client_id).json()
imageUrl = r['urls']['regular']
artist = r['user']['portfolio_url']
#convert to filename
filename = '1.jpg'
img_data = requests.get(imageUrl).content
with open(filename, 'wb') as handler:
handler.write(img_data)
#login to twitter
auth = tweepy.OAuthHandler(keys.twitter_api_key, keys.twitter_api_secret_key)
auth.set_access_token(keys.twitter_access_token, keys.twitter_access_token_secret)
api = tweepy.API(auth)
#create tweet
res = api.media_upload(filename)
api.update_status(status=
"good day from "+place+" \n"
"weather is: "+ str(weather)+"c " + icon + "\n\n"+
"my code: adamsgames.com/twitter\n"+
"weather from: openweathermap.org\n"+
place + " image: " + artist + ", unsplash.com",
media_ids=[res.media_id])
time.sleep(2 * 60 * 60 * 24) # every 2 days